Skip to content
This is simple but most effective routing for reading temperature read from the max31865 and max31856 chip. Hope this might helps some one.
sbit THERMO_CS at PORTD.B5 ;
sbit THERMO_CS_Direction at TRISD5_Bit;
sbit THERMO_CS2 at PORTA.B3 ;
sbit THERMO_CS2_Direction at TRISA3_Bit;
sbit SoftSpi_SDO at RB3_bit;
sbit SoftSpi_SDI at RD4_bit;
sbit SoftSpi_CLK at RB4_bit;
sbit SoftSpi_SDO_Direction at TRISB3_bit;
sbit SoftSpi_SDI_Direction at TRISD4_bit;
sbit SoftSpi_CLK_Direction at TRISB4_bit;
unsigned int rtd_data;
float resistance, temperature;
char txt1[15],text[15];
char msb_v,lsb_v,tempLTHB,tempLTMB,tempLTLB,ID;
unsigned long tempF, tempC;
float rtemp;
void Initialize_Max31856()
{
THERMO_CS2 = 0;
Soft_SPI_Write(0x80);
Soft_SPI_Write(0xCD); //Auto conversion 9A
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x81);
Soft_SPI_Write(0x03); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x82);
Soft_SPI_Write(0xff); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x83);
Soft_SPI_Write(0x7f); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x84);
Soft_SPI_Write(0xc0); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x85);
Soft_SPI_Write(0x7f); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x86);
Soft_SPI_Write(0xff); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x87);
Soft_SPI_Write(0x80); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x89);
Soft_SPI_Write(0x00); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x8A);
Soft_SPI_Write(0x00); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
THERMO_CS2 = 0;
Soft_SPI_Write(0x8B);
Soft_SPI_Write(0x00); //Auto conversion
THERMO_CS2 = 1;
Delay_ms(2);
}
void MAX31856_Read()
{
THERMO_CS2 = 0;
Soft_SPI_Write(0x0C);
tempLTHB = Soft_SPI_Read(0); // Register 0Ch
tempLTMB = Soft_SPI_Read(0); // Register 0Dh
tempLTLB = Soft_SPI_Read(0); // Register 0Eh
THERMO_CS2 = 1;
tempC = 0;
tempC = tempLTHB <<8 | tempLTMB;
tempC = tempC << 3 ;
tempLTLB = tempLTLB >> 5 & 0x07;
lo(tempC) = lo(tempC) | tempLTLB ;
tempC = tempC >> 1 ;
rtemp = tempC * 0.015625 ; // 0.015625; 0.0078125
FloatToStr(rtemp, text);
}
void int_max31865()
{
THERMO_CS = 0;
Soft_SPI_Write(0x80);
Soft_SPI_Write(0xE3);
THERMO_CS = 1;
}
unsigned int max31865_chip_read(unsigned char address)
{
unsigned int value = 0;
THERMO_CS = 0; // Select MAX31865
Soft_SPI_Write(address); // Send register address
value = Soft_SPI_Read(0xFF); // Read register value
value <<= 8;
value |= Soft_SPI_Read(0xFF);
THERMO_CS = 1; // Deselect MAX31865
value >>= 1;
return value;
}
void MAX31865_Read()
{
rtd_data = max31865_chip_read(0x01);
resistance = (float)rtd_data * 390 / 400;
temperature = resistance /32 ;
temperature = temperature – 256;
FloatToStr(temperature, txt1);
}
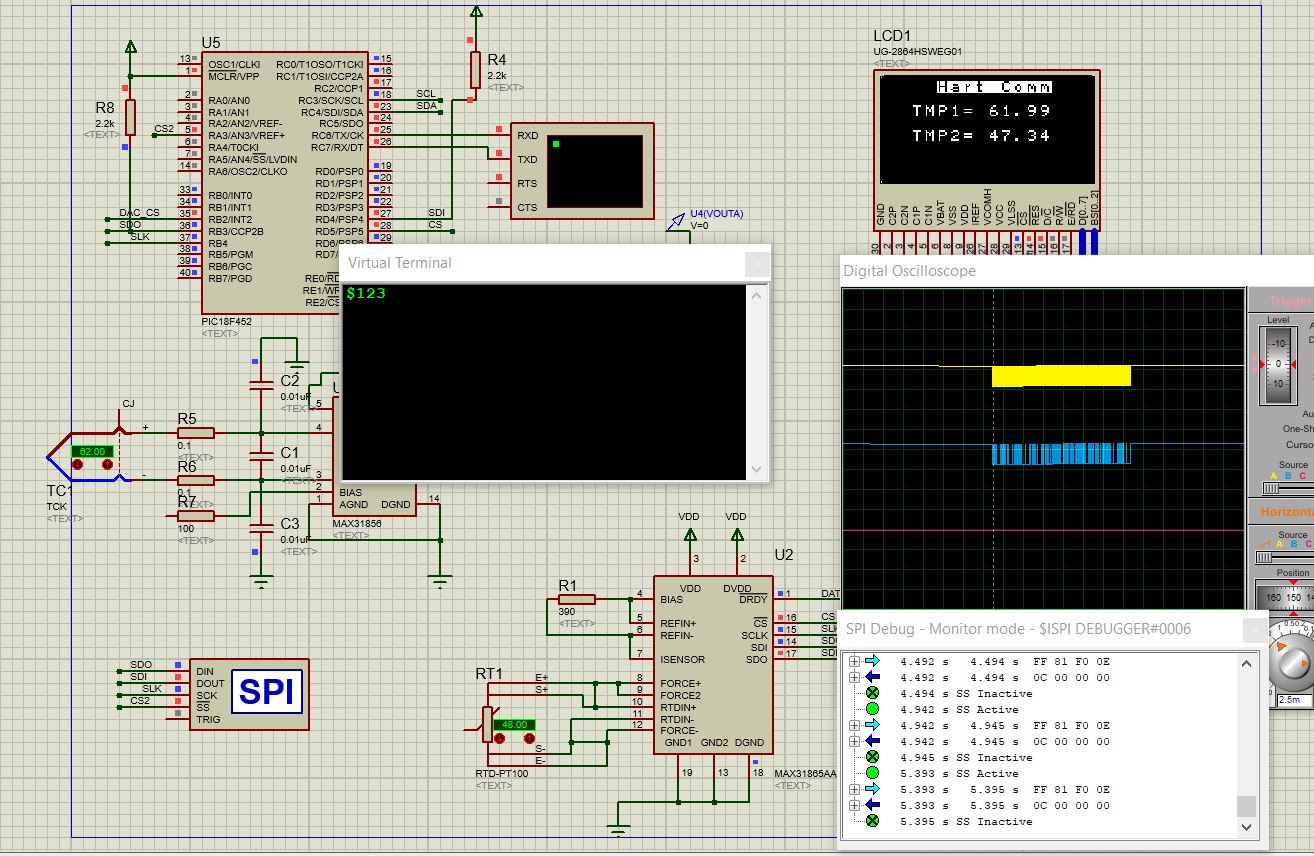
comments are welcome,